Please read this section carefully and thoroughly to understand what parameters you will need to pass.
The SkipSDK.launchSkip function takes in a SkipSDK.Config parameter. This Config parameter sets up how the SDK will behave and has several parameters that must be set. In the following section we will cover each of those parameters and how they affect the functionality of the Skip SDK.
Launch Type
The first thing you need to determine is whether you will be using the Skip map to show nearby stores or your own map. If you are using the Skip map, your launch type will need to be SkipSDKLaunchType.retailer
. If you are using your own map and then launching the Skip experience for a specific store that the customer chooses from your map, then you will need to use the SkipSDKLaunchType.store
.
Intro Type
This parameters affects the customer’s experience the first time they launch the Skip SDK. We need them to accept the Skip Terms of Service and Privacy Policy. We have built a screen that alerts the user of this. For convenience, we have included several different versions of this screen. One version simply tells the customer that they are agreeing to the Skip Terms of Service and Privacy Policy, while others also include a small introduction to what the Skip experience is and what they will be able to use it for. If you are providing your own introduction to the Skip experience, we recommend that you use the SkipIntroType.simple
type. If you are not going to include your own introduction to the Skip experience, we recommend that you use SkipIntroType.custom
, which will allow you to specify custom values for a few key items;
- Program Name (i.e. “Ricker’s Mobile Checkout”, “Jim’s Jiffy Checkout”, “Contact Free Shopping” etc)
- Service 1 or 2 (i.e. “Scan & Go”, “Order Ahead”, “Mobile Ordering”, “Scan & Pay”)
- Examples
- Example 1:
swift SkipIntroType.custom(programName: "Mobile Checkout", features: [SkipIntroFeature(title: "Scan & Go", description: "In-store Self Checkout")], explainer: "You can now use our app to check yourself out quickly & safely!")
- Example 2:
swift SkipIntroType.custom(programName: "Contact Free Checkout", features: [SkipIntroFeature(title: "Scan & Pay", description: "In-store Self Checkout"), SkipIntroFeature(title: "Order Ahead", description: "Curbside or Counter Pick-Up")], explainer: "Shop contact free in our stores or place an order for pick-up and wait for your order at the curb!")
- Example 3:
swift SkipIntroType.custom(programName: "Zippy Checkout", features: [SkipIntroFeature(title: "Scan, Pay, Go", description: "Self Checkout on your phone!")], explainer: "Get in & get out in a Zip!")
- Example 1:
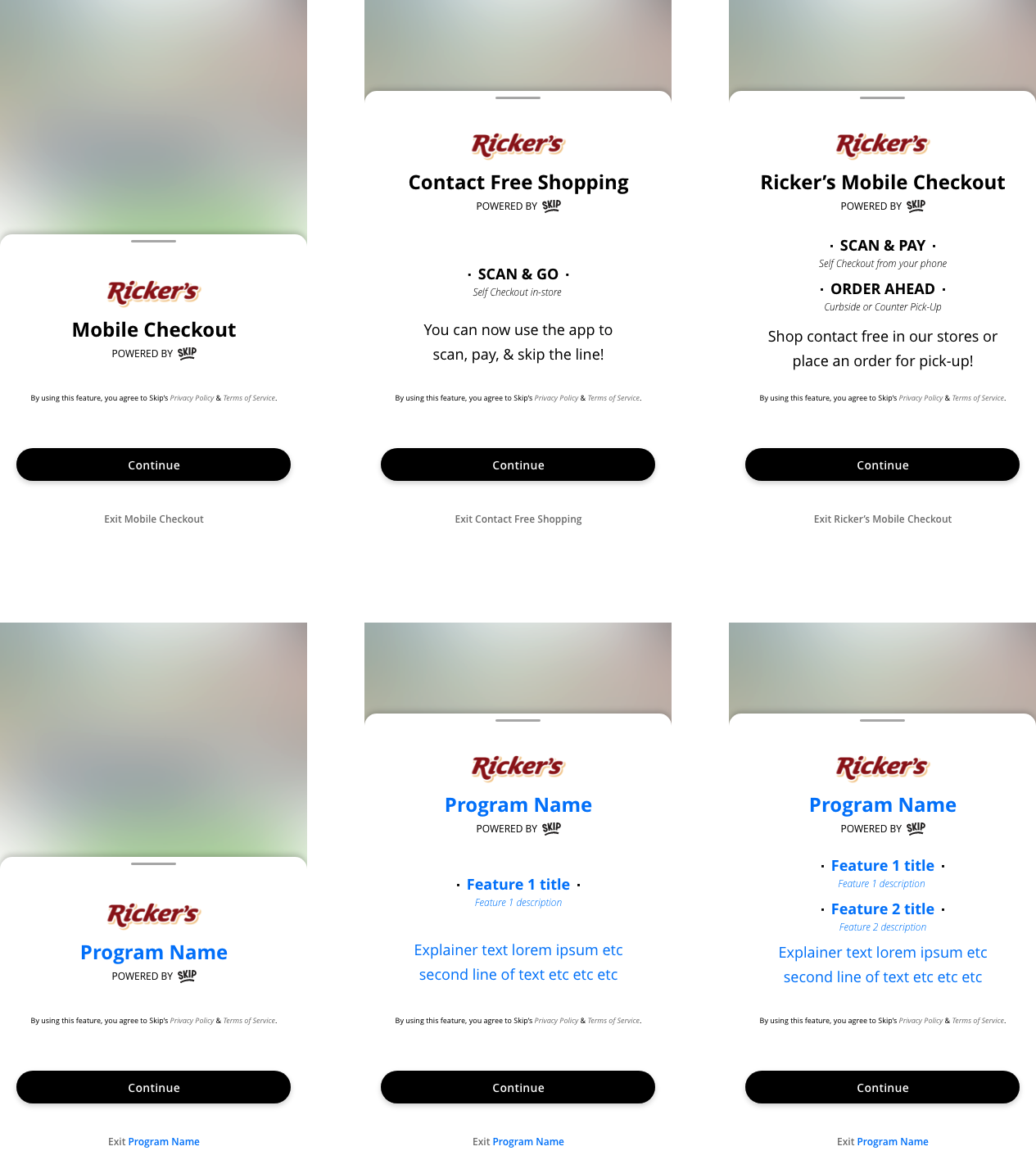
Wallet Type
The next thing you need to determine is who will be handling the wallet. If Skip is handling the wallet and processing payments, then you will need to use SkipSDKWalletType.skipWallet
for the walletType
parameter. If you are the one that will be handling the wallet and processing the payments, then you will need to use the SkipSDKWalletType.providedWallet
for the walletType
parameter.
- Provided Wallet
Your server will need to implement several functions to facilitate being able to start transactions, handle the wallet and report completed transactions. You will need to implement the Skip SDK Callback functions so that we can tell you when to call out to your server to perform these different functions. More information can be found in the Skip SDK Callback section below.
Payment Methods
If you are using the SkipSDKWalletType.skipWallet
type, then you can ignore this parameter.
This allows you to pass in a list of payment methods. These will be formatted as SkipSDKPaymentMethod
objects. You can find out more details about the SkipSDKPaymentMethod
object type below. This parameter is a list of payment methods that allows the shopper to see which payment methods are available to them while using the Skip experience. It will also allow them to select which of these payment methods they would like to use when paying for the Skip transaction. Since you are the one handling the wallet and processing the payment, this is for UX reasons and purely cosmetic. They won’t be able to edit these payment methods within the Skip experience and if they want to add a new payment method, the control will be given back to your app to handle that flow. You can find more details about adding a new payment method from the Skip experience down below in the Skip SDK Callbacks section.
- Apple Pay
If your app allows Apple Pay as a payment method and the customer has Apple Pay enabled on their device, you can specify that by creating a SkipSDKPaymentMethod and setting the type asSkipSDKPaymentType.applePay
.
Shopper Type
Next you will need to determine the shopper type. There are two types, SkipSDKShopperType.skipShopper
and SkipSDKShopperType.anonymous
shoppers. If Skip is handling the wallet and processing the payments, then you need to use SkipSDKShopperType.skipShopper
as the shopperType
parameter. If you are handling the wallet and processing the payments, you have to use SkipSDKShopperType.anonymous
shopper type. There are benefits that come with using Skip Shoppers. These benefits include the shopper being able to see past receipts, recently purchased items, frequently purchased items, storing loyalty cards, etc.
- SkipShopper
If you are using the SkipShopper type, the customer will have to “login” to the skip platform. To make this process as seamless as possible, you’re able to pass existing information that you have about the shopper. This includes first and last name, email and phone number. The phone number must match the E.164 plan.
Essentially the phone number should be in the format+1(area code)(phone number) i.e. +12223334444
If all four of those data points are passed in, the customer will automatically be signed up and logged in on the Skip platform the first time they launch the Skip experience. They will stay logged in as long as the customer doesn't clear your app’s data or uninstall your app from their device. To pass in this information about the customer, you’ll create aSkipSDKUser
object and pass the known information in the constructor. You can find a more detailed example below.
Order Ahead
Order Ahead is currently only available if you are using SkipSDKWalletType.skipWallet
NOT SkipSDKWalletType.providedWallet
.
- If you are using the Skip map, shoppers will already be able to select between Order Ahead or Scan and Go shopping trip from the Skip map experience.
- If you are using the
SkipSDKLaunchType.store
andSkipSDKShopperType.skipShopper
type, you can choose between a Scan and Go or Order Ahead shopping trip type. To do this, you will specify the shopping trip type within theSkipSDKLaunchType.store
parameter. This shopping trip type will default to Scan and Go if no parameter is passed.
SkipSDKLaunchType.store(storeId: [insert store Id], retailerId: [insert retailer Id], SkipSDKShoppingTripType.orderAhead)
Note: You should use a store Id and Retailer Id that is provided to you by the Skip Product Manager assisting with your implementation. Please contact Skip for a store and retailer Id, or list of Store IDs to use.
Loyalty
If Skip is integrated with the loyalty provider for your retailer, and you have the loyalty ID for your customer, you can pass that through inside the Loyalty parameter and we will attempt to link that loyalty number to the cart for this shopping trip.
Environment
We recommend doing your development in the sandbox environment. This ensures that you will not be charged for any completed transactions if you’re using the Skip Wallet and letting Skip process the payments. To specify the environment, you can set the environment parameter when launching the Skip experience.
You will need to be sure that this parameter is set to SkipSDKEnvironment.production
when you’re releasing your application to the public.
Skip SDK Callbacks
This is the bridge that allows us to communicate with your app while the Skip experience is running or to let you know that the Skip experience is about to close.
-
Skip Wallet: If you are using the
SkipSDKWalletType.skipWallet
type, this parameter is optional. The only function that might be important for you to implement is theskipWillClose
function. This function allows us to notify you that the Skip experience is about to close. It includes a parameter that tells you whether the customer completed their shopping trip or terminated the Skip experience before they were done shopping. -
Provided Wallet: If you are using the
SkipSDKWalletType.providedWallet
type, this parameter is required. There are several functions that you need to implement to facilitate communication between your app and the Skip experience.startSkipCart
tenderSkipCart
addNewPaymentMethodCalledFromSkipExperience
startSkipCart is used to alert your app that the shopper would like to start a Skip cart. We will tell you which store ID to start the cart in. You will then need to call your server and tell them to start a skip cart for the provided store ID. When they receive a successful response they will need to pass the response back to you so that you can pass it through to the SDK in the provided completionHandler.
tenderSkipCart is used to alert your app that the shopper is ready to tender the Skip cart. If you have provided multiple payment methods to the Skip SDK, the shopper has the option within the Skip experience to choose from those payment methods which one they would like to use to pay with. We will tell you how much to tender the cart for and which payment method the Shopper has chosen to pay with. You need to call your server to tender the cart for the provided amount. Once your server has tendered the cart, they will alert our server that it has been tendered, and our server will alert the Skip SDK that the cart has been tendered.
addNewPaymentMethodCalledFromSkipExperience is used to alert your app that the shopper would like to add a new payment method. Because you are the one handling the wallet, you will then have control to show the user your flow for adding a payment method and the Skip SDK will be suspended in the background. Once the user is done adding that new payment method, you will need to call the
SkipUISDK.updatePaymentMethods
function with the updated list of payment methods that should include the new one the shopper just added to your app.skipWillClose allows us to notify you that the Skip experience is about to close. It includes a parameter that tells you whether the customer completed their shopping trip or terminated the Skip experience before they were done shopping.